Let’s see how you can filter TArray of Actors with multiple Class choices. We want to filter Actor by the class.
There’s kismet array library which you need to include:
#include "Kismet/KismetArrayLibrary.h"
There’s UKismetMathLibrary::ClassIsChildOf function too but for some reason couldn’t get that to work, so let’s use what there already is.
There’s Filter Array but it only pops up if you drag from array and it only has single filter option. We are looking to filter by multiple choices so this is not good enough.
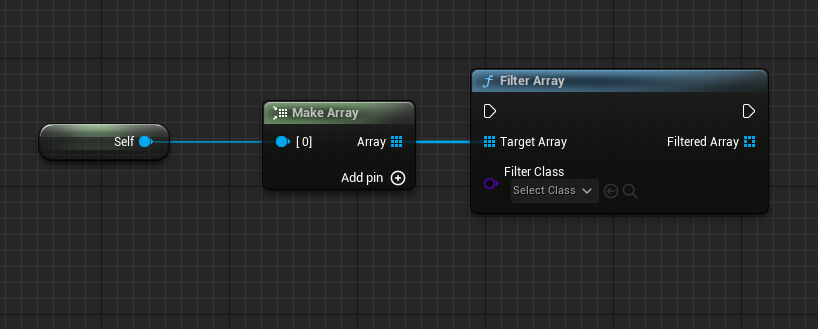
And here’s the code. Create blueprint function library Class and add it in there.
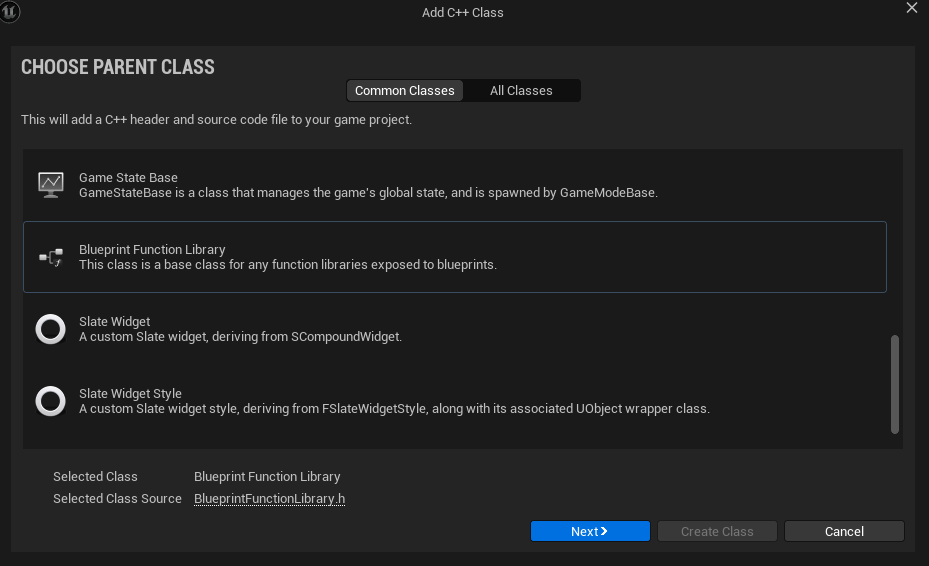
public:
/* Leave your comment
*/
UFUNCTION(BlueprintPure, Category = "My Custom Blueprint Function Library")
static TArray<AActor*> ActorClassFilter(TArray<AActor*> Actors, TArray<TSubclassOf<AActor>> Filter);
You can also change TArray<TSubclassOf<AActor>> Filter to this: TArray<UClass*> Filter and it will work.
TArray<AActor*> UMyBlueprintFunctionLibrary::ActorClassFilter(TArray<AActor*> Actors, TArray<TSubclassOf<AActor>> Filter)
{
TArray<AActor*> tmp;
for (auto FilterItem : Filter)
{
TArray<AActor*> save;
UKismetArrayLibrary::FilterArray(Actors, FilterItem, save);
tmp += save;
}
return tmp;
}
Result will look like this:
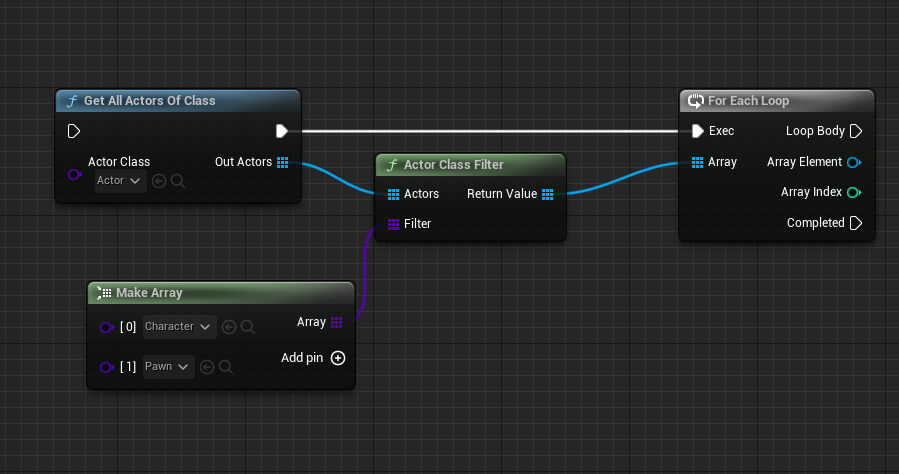