In this tutorial we will turn this blueprint graph below into C++.
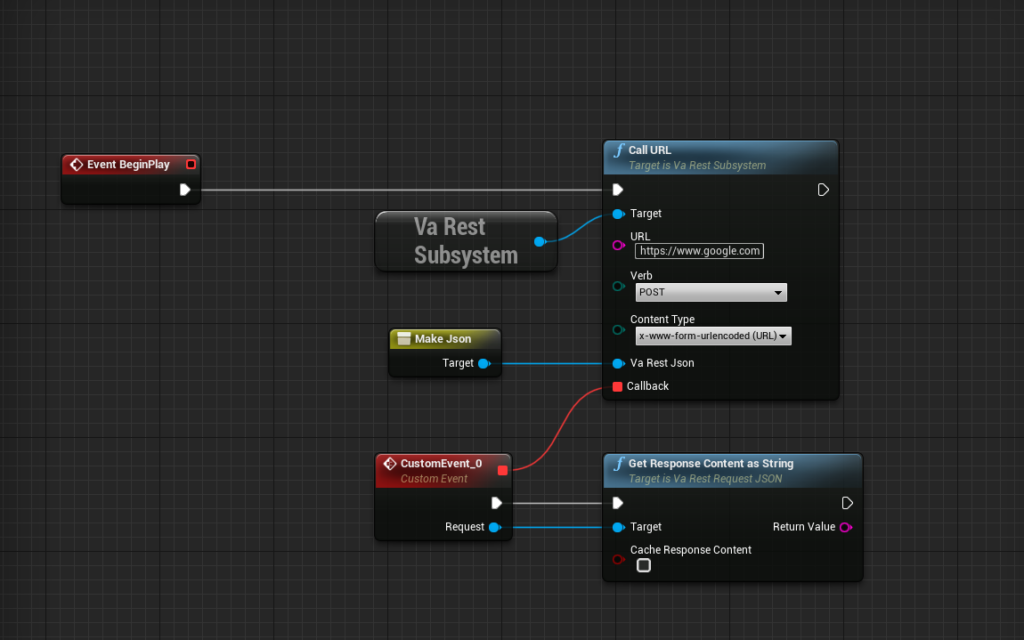
Step 1
You can either download VaRest from github or from Unreal Marketplace.
Download the proper VaRest version for your Project:
https://github.com/ufna/VaRest
Add the files to your Project Plugins Folder:
MyProject/Plugins/VaRest
If you use marketplace just jump to step 2
Step 2
Add VaRest module to MyProject.Build.cs File.
using UnrealBuildTool;
public class CHANGETHIS: ModuleRules
{
public CHANGETHIS(ReadOnlyTargetRules Target) : base(Target)
{
PCHUsage = PCHUsageMode.UseExplicitOrSharedPCHs;
PublicDependencyModuleNames.AddRange(new string[] { "Core", "CoreUObject", "Engine", "InputCore", "VaRest" });
PrivateDependencyModuleNames.AddRange(new string[] { });
}
}
Step 3
Time to add some code. I’m using AActor Class.
So first we add some headers into x.h file
#include "VaRestSubsystem.h"
#include "VaRestRequestJSON.h"
#include "VaRestTypes.h"
#include "VaRestJsonObject.h"
#include "VaRestJsonValue.h"
Then we add VaRest Delegate
FVaRestCallDelegate VarestDelegate;
Then comes delegate result function.
Always add UFUNCTION into this or it wont be called by delegate.
void VaRestTest(UVaRestRequestJSON* Request);
This we use to start whole thing
void VaRestInit(FString URL);
Function that gets the subsystem
UVaRestSubsystem* GetVARestSub();
And whole thing.
#pragma once
#include "CoreMinimal.h"
#include "VaRestSubsystem.h"
#include "VaRestRequestJSON.h"
#include "VaRestTypes.h"
#include "VaRestJsonObject.h"
#include "VaRestJsonValue.h"
#include "Subsystems/SubsystemBlueprintLibrary.h"
#include "GameFramework/Actor.h"
#include "ResTest.generated.h"
UCLASS()
class AResTest : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
AResTest();
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
virtual void Tick(float DeltaTime) override;
FVaRestCallDelegate VarestDelegate;
//UFUNCTION is really important here
UFUNCTION(BlueprintCallable, Category = "Tests")
void VaRestTest(UVaRestRequestJSON* Request);
UFUNCTION(BlueprintCallable, Category = "Tests")
void VaRestInit(FString URL);
UFUNCTION(BlueprintCallable, Category = "Tests")
UVaRestSubsystem* GetVARestSub();
};
#include "ResTest.h"
// Sets default values
AResTest::AResTest()
{
// Set this actor to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = true;
}
// Called when the game starts or when spawned
void AResTest::BeginPlay()
{
Super::BeginPlay();
}
// Called every frame
void AResTest::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}
//Don't add any other parameters into this just what the VaRestDelegate gives
void AResTest::VaRestTest(UVaRestRequestJSON* Request)
{
FString Response = Request->GetResponseContentAsString(false);
FString Message = FString();
Message.Append(TEXT("Request went through"));
Message.Append(TEXT(" Response Length: "));
Message.Append(FString::FromInt(Response.Len()));
GEngine->AddOnScreenDebugMessage(-1, 9.f, FColor::Yellow, Message);
}
void AResTest::VaRestInit(FString URL)
{
UVaRestSubsystem* Varestsub = GetVARestSub();
if (!::IsValid(Varestsub))
{
GEngine->AddOnScreenDebugMessage(-1, 4.f, FColor::Red, TEXT("VArest Subsystem missing"));
return;
}
//This will bind VaRestTest function to VarestDelegate, which is how you get the result from CallUrl
VarestDelegate.BindUFunction(this, FName("VaRestTest"));
//This is the Call Url Function
Varestsub->UVaRestSubsystem::CallURL(URL, EVaRestRequestVerb::POST, EVaRestRequestContentType::x_www_form_urlencoded_url, ((UVaRestJsonObject*)nullptr), VarestDelegate);
}
UVaRestSubsystem* AResTest::GetVARestSub()
{
UVaRestSubsystem* Subsystem{};
Subsystem = CastChecked<UVaRestSubsystem>(USubsystemBlueprintLibrary::GetEngineSubsystem(UVaRestSubsystem::StaticClass()), ECastCheckedType::NullAllowed);
if (::IsValid(Subsystem))
{
return Subsystem;
}
return nullptr;
}
And that’s it, edit it into your needs, good luck!
If you need to multithread this task check out this: